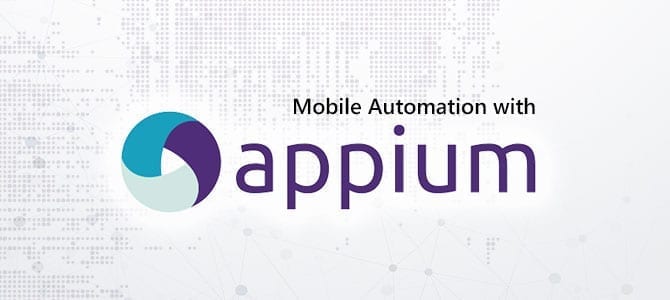
XPath is a powerful language for navigating through elements and attributes in an XML document. In the context of Appium, XPath is an essential tool for locating UI elements within mobile applications. This blog will explore the usage of XPath in Appium test code through a detailed use case, demonstrating how to effectively write XPath expressions to automate tests.
What is XPath?
XPath, short for XML Path Language, is a query language used to select nodes from an XML document. In mobile test automation, XPath is used to locate elements in the XML representation of the app's UI. XPath provides a flexible and powerful way to identify elements based on various attributes, positions, and hierarchical relationships. Need testing? – Try RobotQA and Start Testing on Real Devices. Start Free Trial
Why Use XPath in Appium?
- Flexibility: XPath allows you to locate elements based on a wide range of criteria, such as tag names, attributes, text content, and hierarchical relationships.
- Complex Scenarios: XPath can handle complex scenarios where other locator strategies, like ID or class name, might fail.
- Dynamic Elements: XPath is useful for locating dynamic elements that change their attributes during runtime.
Use Case: Automating a Login Test
Let's consider a use case where we need to automate the login process of a mobile application. The login screen contains the following elements:- Username field
- Password field
- Login button
Step 1: Setting Up the Environment
Ensure you have the necessary setup for Appium, including the Appium server, Appium Desktop (for inspecting elements), and the desired capabilities configured for your Android device.Step 2: Inspecting Elements Using Appium Inspector
- Launch Appium Server and Appium Inspector.
- Start a session with the app running on your device.
- Inspect the elements on the login screen.
- Username field:
//android.widget.EditText[@resource-id='com.example:id/username']
- Password field:
//android.widget.EditText[@resource-id='com.example:id/password']
- Login button:
//android.widget.Button[@text='Login']
Step 3: Writing the Test Code
Using the XPaths identified, let's write the Appium test code in Java to automate the login process.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
import io.appium.java_client.MobileElement; import io.appium.java_client.android.AndroidDriver; import io.appium.java_client.remote.MobileCapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import java.net.URL; public class LoginTest { public static void main(String[] args) { DesiredCapabilities caps = new DesiredCapabilities(); caps.setCapability(MobileCapabilityType.PLATFORM_NAME, "Android"); caps.setCapability(MobileCapabilityType.PLATFORM_VERSION, "11.0"); caps.setCapability(MobileCapabilityType.DEVICE_NAME, "YourDeviceName"); caps.setCapability(MobileCapabilityType.APP, "/path/to/your/app.apk"); caps.setCapability(MobileCapabilityType.AUTOMATION_NAME, "UiAutomator2"); try { AndroidDriver<MobileElement> driver = new AndroidDriver<>(new URL("http://localhost:4723/wd/hub"), caps); // Locate and interact with the Username field MobileElement usernameField = driver.findElementByXPath("//android.widget.EditText[@resource-id='com.example:id/username']"); usernameField.sendKeys("testuser"); // Locate and interact with the Password field MobileElement passwordField = driver.findElementByXPath("//android.widget.EditText[@resource-id='com.example:id/password']"); passwordField.sendKeys("password123"); // Locate and click the Login button MobileElement loginButton = driver.findElementByXPath("//android.widget.Button[@text='Login']"); loginButton.click(); // Additional steps to verify login success can be added here driver.quit(); } catch (Exception e) { e.printStackTrace(); } } } |
Tips for Writing Effective XPath Expressions
- Use Unique Attributes: Whenever possible, use unique attributes like
resource-id
orcontent-desc
to locate elements.1MobileElement element = driver.findElementByXPath("//android.widget.Button[@content-desc='loginButton']"); - Index-Based Selection: If elements are in a list or grid, you can use indexing to select a specific element.
1MobileElement firstItem = driver.findElementByXPath("(//android.widget.TextView)[1]");
- Hierarchical Relationships: Use the hierarchical structure of elements to locate them relative to their parent or sibling elements.
1MobileElement element = driver.findElementByXPath("//android.widget.LinearLayout/android.widget.TextView[@text='Settings']");
- Text-Based Selection: Locate elements based on their text content.
1MobileElement element = driver.findElementByXPath("//android.widget.TextView[@text='Submit']");
- Contains Function: Use the
contains
function to match partial text or attribute values.1MobileElement element = driver.findElementByXPath("//android.widget.TextView[contains(@text, 'Log')]");