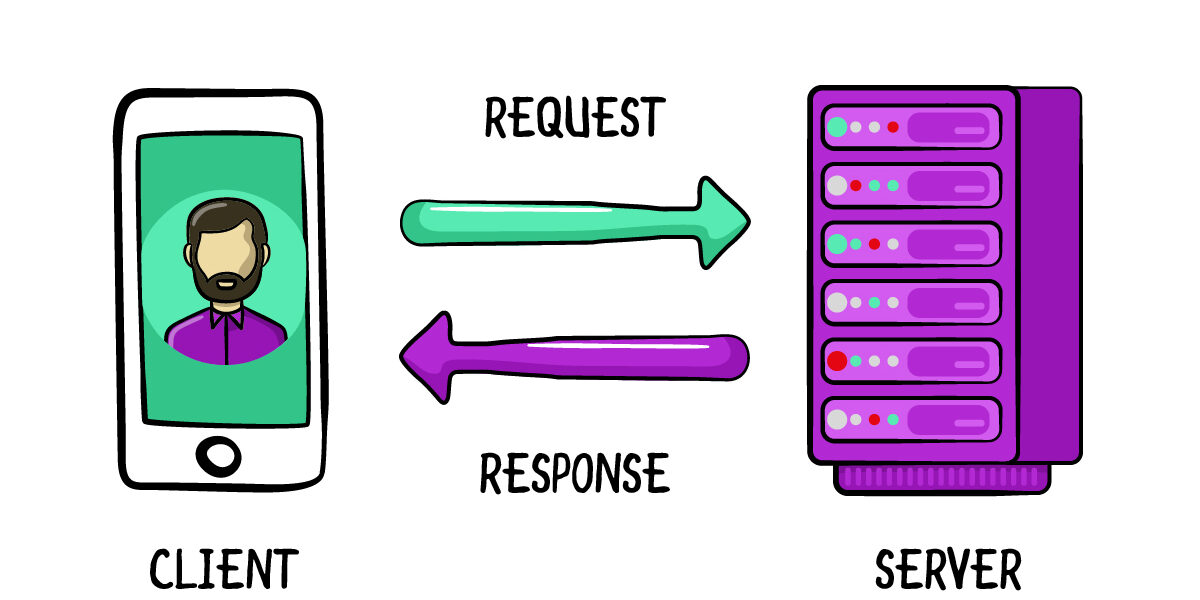
Best 5 iOS HTTP Client Third-Party Tools
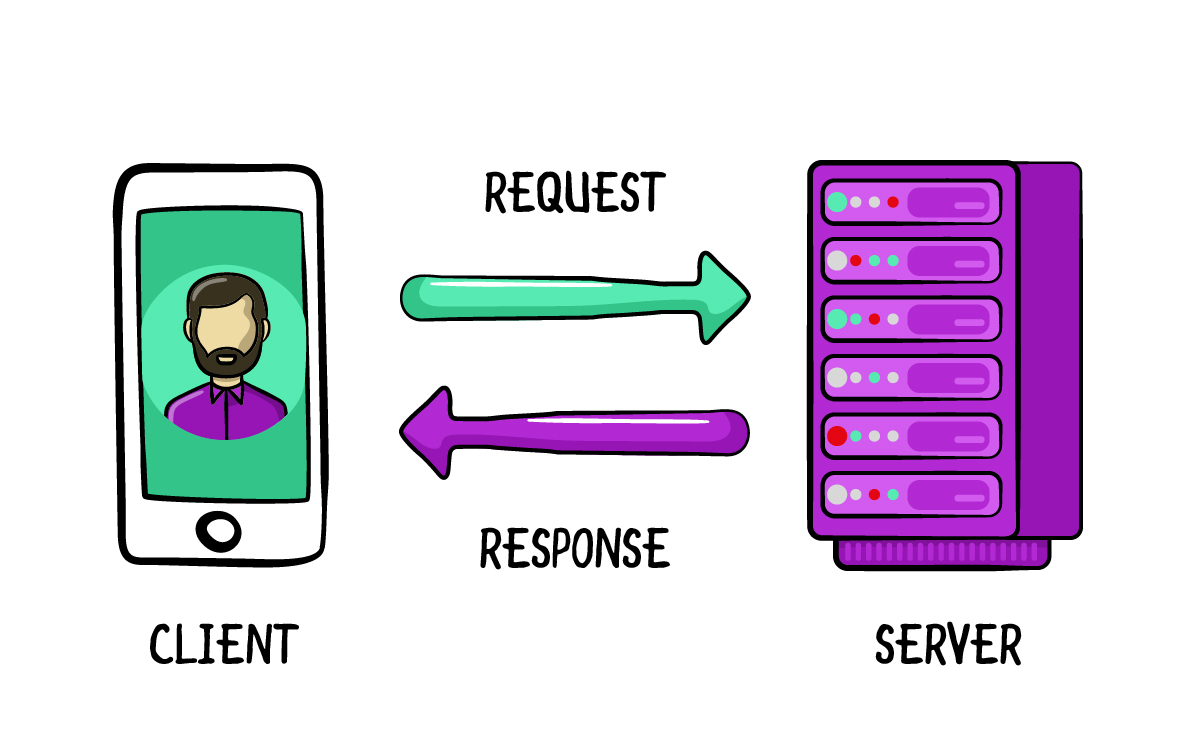
1. Alamofire
Overview
Alamofire is the most popular Swift-based HTTP networking library for iOS. It provides an elegant interface to handle network requests, making it a favorite among iOS developers.Key Features
- Chainable Request/Response Methods: Simplifies complex HTTP operations with chainable methods.
- JSON Parsing: Easily decode JSON responses using Codable.
- Request Retrying: Built-in mechanisms for retrying failed requests.
- Authentication: Supports various authentication methods, including OAuth.
- Network Reachability: Monitor network status and respond to changes.
Example
Alamofire.request("https://api.example.com/data") .validate() .responseJSON { response in switch response.result { case .success(let value): print("JSON: \(value)") case .failure(let error): print("Error: \(error)") } }
Use Case
Ideal for apps requiring sophisticated networking with easy JSON handling and complex request chaining.2. AFNetworking
Overview
AFNetworking is a powerful and flexible networking library for Objective-C. While Alamofire has become more popular with the rise of Swift, AFNetworking remains a solid choice for Objective-C projects.Key Features
- JSON, XML, and Property List Response Serializers: Handles multiple data formats.
- Image Response Serializer: Useful for image-heavy applications.
- Network Reachability: Monitor network connectivity and respond accordingly.
- Security: Supports SSL pinning and other security features.
Example
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager]; [manager GET:@"https://api.example.com/data" parameters:nil progress:nil success:^(NSURLSessionTask *task, id responseObject) { NSLog(@"JSON: %@", responseObject); } failure:^(NSURLSessionTask *operation, NSError *error) { NSLog(@"Error: %@", error); }];
Use Case
Perfect for Objective-C applications that need robust networking features and strong security. Need Debugging? – Try RobotQA and Start Debugging on Real Devices. Download Plugin
3. Moya
Overview
Moya is a network abstraction layer that builds on top of Alamofire, offering a more structured approach to network requests by defining them as part of an enum.Key Features
- API Abstraction: Encapsulates API details, making code more maintainable.
- Plugins: Extensible through plugins for logging, network activity indicators, etc.
- Stubbing: Easily stub out network responses for testing.
Example
let provider = MoyaProvider<MyService>() provider.request(.getData) { result in switch result { case .success(let response): print("Response: \(response)") case .failure(let error): print("Error: \(error)") } }
Use Case
Great for projects that require clear separation of network logic from the rest of the app, promoting better organization and testability.4. URLSession
Overview
URLSession is Apple’s native networking API, providing a robust foundation for making HTTP requests. While not a third-party library, it is often used in conjunction with other tools to handle low-level network tasks.Key Features
- Data Tasks, Download Tasks, and Upload Tasks: Versatile task handling for various network operations.
- Background Sessions: Supports background downloads and uploads.
- Configurable: Highly configurable for caching, timeout, and other parameters.
- Security: Strong support for HTTPS, SSL pinning, and authentication.
Example
let url = URL(string: "https://api.example.com/data")! let task = URLSession.shared.dataTask(with: url) { data, response, error in if let data = data { print("Data: \(data)") } else if let error = error { print("Error: \(error)") } } task.resume()
Use Case
Ideal for developers who need fine-grained control over network operations and prefer using Apple’s built-in frameworks.5. Siesta
Overview
Siesta is a Swift framework that provides a powerful and flexible way to manage the lifecycle of network requests and responses, emphasizing simplicity and ease of use.Key Features
- Resource-centric API: Abstracts away URL requests into resources, simplifying state management.
- Automatic Caching: Built-in caching mechanisms to improve performance.
- Observable Resources: Easily observe changes to resources for reactive programming.
- Error Handling: Comprehensive error handling and retry logic.
Example
let api = Service(baseURL: "https://api.example.com") let resource = api.resource("/data") resource.addObserver(owner: self) { resource, event in if let data = resource.latestData { print("Data: \(data)") } } resource.loadIfNeeded()