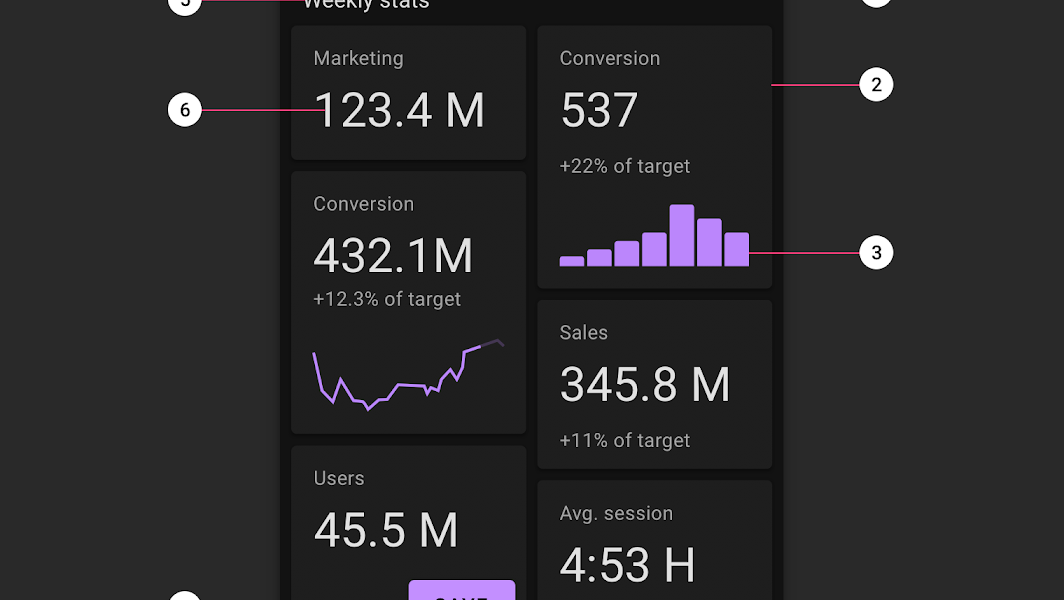
Creating a Modern UI with Material Dark Theme in Android
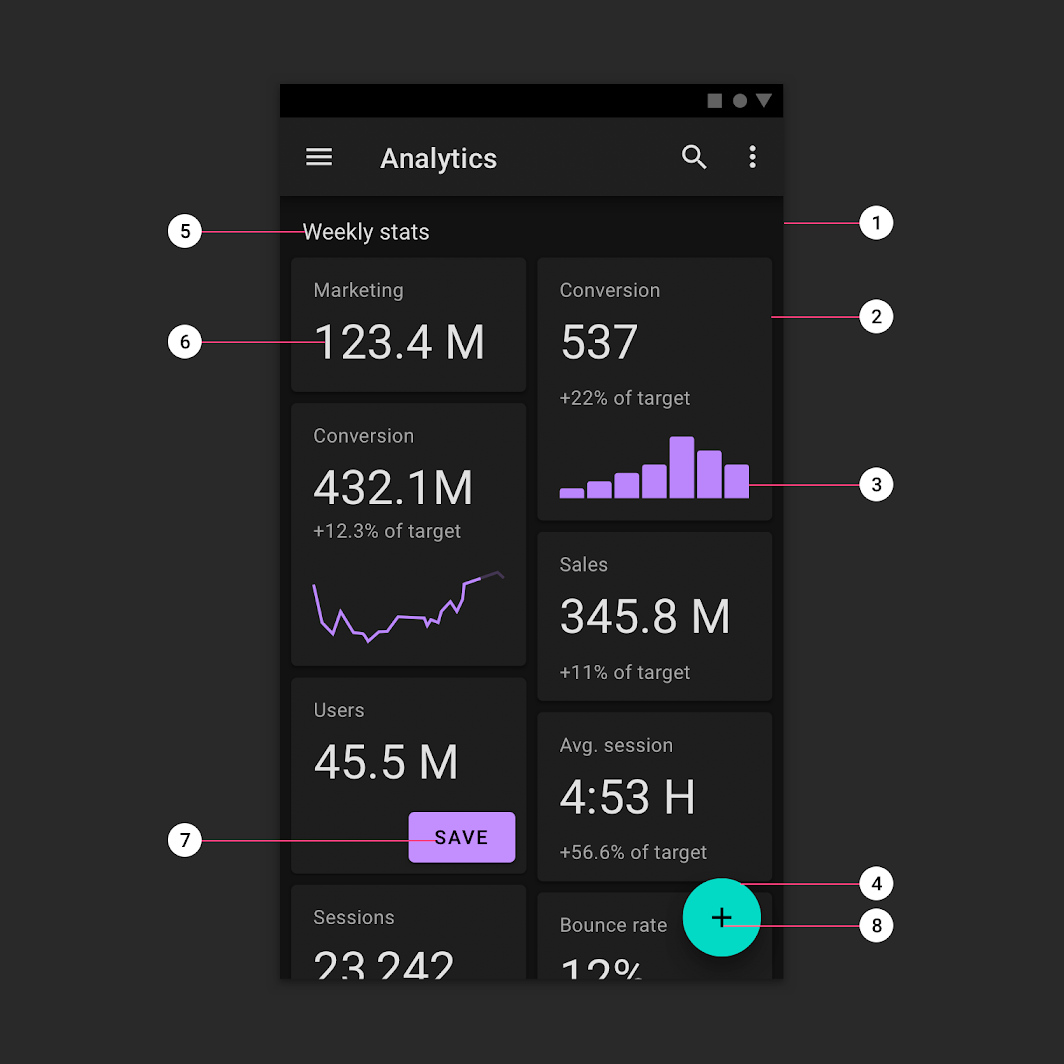
Setting Up the Material Dark Theme
First, ensure you have the necessary dependencies in yourbuild.gradle
file.
Step 1: Add Dependencies
Open yourbuild.gradle
file and add the Material Components dependency:
dependencies { implementation 'com.google.android.material:material:1.8.0' implementation 'androidx.appcompat:appcompat:1.3.1' implementation 'androidx.core:core-ktx:1.6.0' }
Step 2: Define the Theme in styles.xml
Open yourres/values/styles.xml
file and define the Material Dark theme:
<resources> <!-- Base application theme --> <style name="AppTheme" parent="Theme.MaterialComponents.DayNight.DarkActionBar"> <!-- Customize your theme here --> <item name="colorPrimary">@color/purple_500</item> <item name="colorPrimaryDark">@color/purple_700</item> <item name="colorAccent">@color/teal_200</item> </style> </resources>
Example: Creating a Simple UI with Material Dark Theme
Let’s create a simple UI that includes a welcome message and a button, all styled with the Material Dark theme.Step 3: Define the Layout
Create an XML layout file (activity_main.xml
) with the following content:
<com.google.android.material.textfield.TextInputLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="16dp"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/inputEditText" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Enter text" android:inputType="text" /> <com.google.android.material.button.MaterialButton android:id="@+id/submitButton" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Submit" android:layout_marginTop="16dp" app:cornerRadius="8dp" android:backgroundTint="@color/teal_200" android:textColor="@android:color/black" /> </com.google.android.material.textfield.TextInputLayout>
Step 4: Initialize in Activity
In yourMainActivity.java
or MainActivity.kt
file, initialize the views and add any necessary functionality.
Java
package com.example.materialdarktheme; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import com.google.android.material.button.MaterialButton; import com.google.android.material.textfield.TextInputEditText; import com.google.android.material.textfield.TextInputLayout; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); TextInputLayout textInputLayout = findViewById(R.id.inputEditText); TextInputEditText textInputEditText = findViewById(R.id.inputEditText); MaterialButton submitButton = findViewById(R.id.submitButton); submitButton.setOnClickListener(v -> { String inputText = textInputEditText.getText().toString(); if (inputText.isEmpty()) { Toast.makeText(MainActivity.this, "Please enter some text", Toast.LENGTH_SHORT).show(); } else { // Handle the input text Toast.makeText(MainActivity.this, "You entered: " + inputText, Toast.LENGTH_SHORT).show(); } }); } }
Kotlin
package com.example.materialdarktheme import android.os.Bundle import android.widget.Toast import androidx.appcompat.app.AppCompatActivity import com.google.android.material.button.MaterialButton import com.google.android.material.textfield.TextInputEditText import com.google.android.material.textfield.TextInputLayout class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val textInputLayout: TextInputLayout = findViewById(R.id.inputEditText) val textInputEditText: TextInputEditText = findViewById(R.id.inputEditText) val submitButton: MaterialButton = findViewById(R.id.submitButton) submitButton.setOnClickListener { val inputText = textInputEditText.text.toString() if (inputText.isEmpty()) { Toast.makeText(this, "Please enter some text", Toast.LENGTH_SHORT).show() } else { // Handle the input text Toast.makeText(this, "You entered: $inputText", Toast.LENGTH_SHORT).show() } } } }
Need Debugging? – Try RobotQA and Start Debugging on Real Devices. Download Plugin